Positive ratings and reviews are essential to ranking higher in the app stores and convincing potential users to install our app.
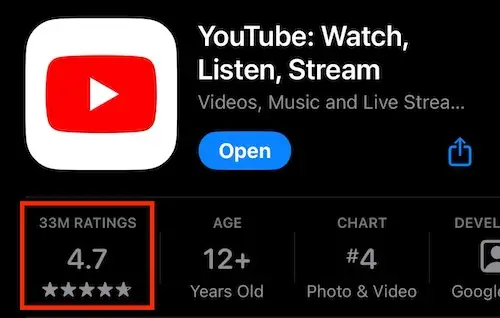
A great way to get more ratings for your app is to directly ask your users using an in-app rating prompt.
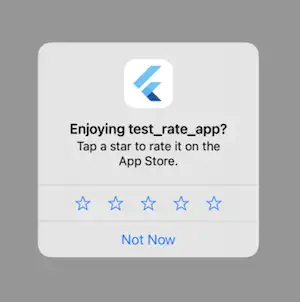
My favorite package for adding a rating prompt to my apps is rate_my_app.
This package is great because it lets us define parameters like minDays
and minLaunches
that the user must meet before displaying the rating prompt. We can also set up remindDays
and remindLaunches
to re-prompt the user after a certain number of days and additional launches.
final _rateMyApp = RateMyApp(
minDays: 1,
minLaunches: 2,
remindDays: 1,
remindLaunches: 2,
appStoreIdentifier: '<app_store_id>',
googlePlayIdentifier: '<google_play_id>',
);
await _rateMyApp.init();
If we use the showStarRateDialog
, we can also take certain actions based on the rating a user gives us.
_rateMyApp.showStarRateDialog(
context,
title: 'Rate App',
message: "If you're enjoying the app, please leave a rating to support us!",
actionsBuilder: (context, stars) {
return [
TextButton(
child: const Text('OK'),
onPressed: () async {
// Example: Launch app store if user rates 5 stars.
if (stars?.round().toString() == '5') {
await _rateMyApp.launchStore();
}
await _rateMyApp.callEvent(RateMyAppEventType.rateButtonPressed);
if (context.mounted) {
Navigator.pop<RateMyAppDialogButton>(
context,
RateMyAppDialogButton.rate,
);
}
},
),
];
},
);
To show the native app review dialog prompt, we have to first check if the native review dialog is supported and then launch the native review dialog.
if (await _rateMyApp.isNativeReviewDialogSupported ?? false) {
_rateMyApp.launchNativeReviewDialog();
}
Here’s a full example of implementing the rate_my_app package with get_it for dependency injection.
// === app_locator.dart ===
/// Global [GetIt.instance].
final locator = GetIt.instance;
/// Set up [GetIt] locator.
void setUpLocator() {
locator.registerSingleton(RateAppHelper());
}
// === rate_app_helper.dart ===
class RateAppHelper {
RateAppHelper({
RateMyApp? rateMyApp,
}) : _rateMyApp = rateMyApp ??
RateMyApp(
minDays: 1,
minLaunches: 2,
remindDays: 1,
remindLaunches: 2,
appStoreIdentifier: '<app_store_id>',
googlePlayIdentifier: '<google_play_id>',
) {
initialize();
}
bool _isNativeReviewDialogSupported = false;
final RateMyApp _rateMyApp;
Future<void> initialize() async {
await _rateMyApp.init();
_isNativeReviewDialogSupported =
await _rateMyApp.isNativeReviewDialogSupported ?? false;
}
// Show native review dialog or standard.
void showDialog(BuildContext context) {
if (_rateMyApp.shouldOpenDialog) {
if (UniversalPlatform.isIOS && _isNativeReviewDialogSupported) {
_rateMyApp.launchNativeReviewDialog();
} else if (UniversalPlatform.isAndroid) {
_rateMyApp.showStarRateDialog(
context,
title: 'Rate App',
message: "If you're enjoying the app, please leave a rating to support us!",
actionsBuilder: (context, stars) {
return [
TextButton(
child: const Text('OK'),
onPressed: () async {
// Launch store if user rates 5 stars.
if (stars?.round().toString() == '5') {
await _rateMyApp.launchStore();
}
await _rateMyApp.callEvent(RateMyAppEventType.rateButtonPressed);
if (context.mounted) {
Navigator.pop<RateMyAppDialogButton>(
context,
RateMyAppDialogButton.rate,
);
}
},
),
];
},
);
}
}
}
}
// === main.dart ===
Future<void> main() async {
WidgetsFlutterBinding.ensureInitialized();
setUpLocator();
runApp(const App());
}
Now, whenever we want a user to review our app, we can use locator
to access the instance of RateAppHelper
and show the dialog!
locator.get<RateAppHelper>().showDialog(context);
Once the user rates the app, the dialog will not show up again. This is taken care of automatically, so there’s no need to add additional logic.